CA-Midterm_1-Review
Midterm_1 Review
-
- 2.1. C Compilation Simplified Overview
- 2.2. Pointer
- 2.3. Array
- 2.3.1. Further explanations:
- 2.3.2. Point past end of array
OverAll
1. Floating point number
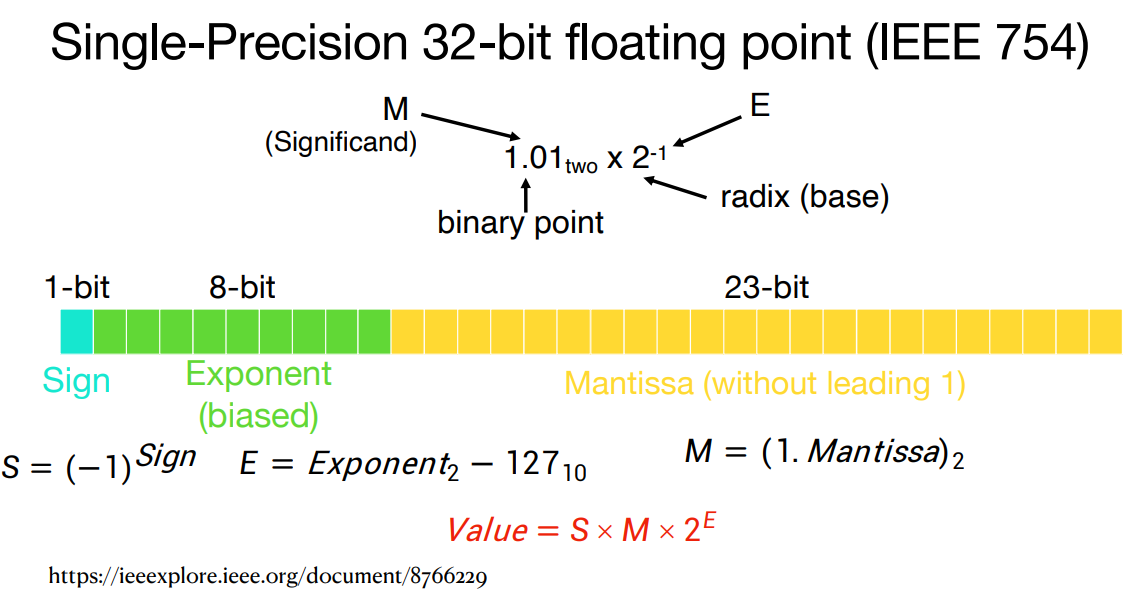
$$
\begin{align}
&S = (-1)^{\text{Sign}}\\
&E = \text{Exponent}_2-127_{10}\\
&Value = S\times M\times2^E
\end{align}
$$
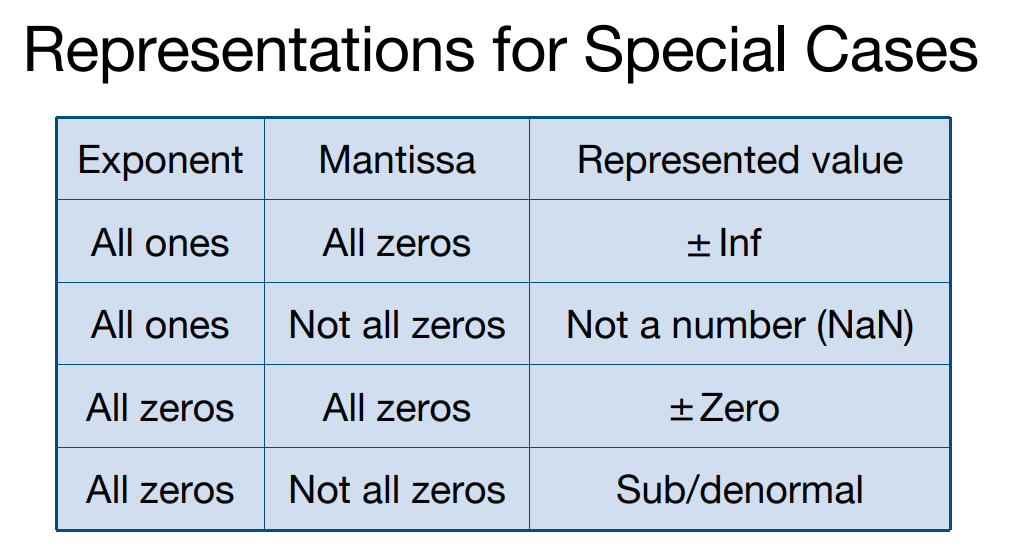
Tips:
Abt denorms: Normalization and hidden 1 is to blame!
The exponent of denorms: $E := -126$
2. How C works?
2.1. C Compilation Simplified Overview
- Generally a two part process of compiling
.c
files to.o
files, then linking the.o
files into executables; - Assembling is also done (but is hidden, i.e., done automatically, by default);
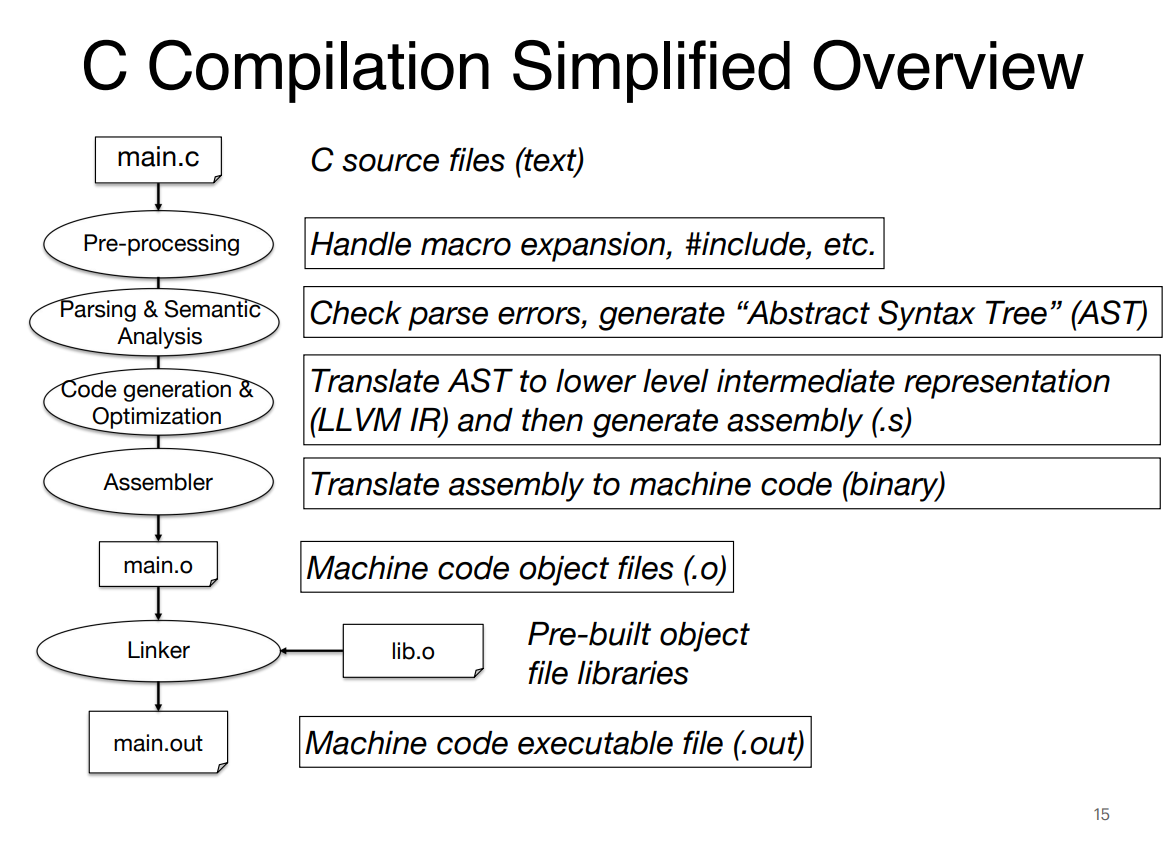
E.G
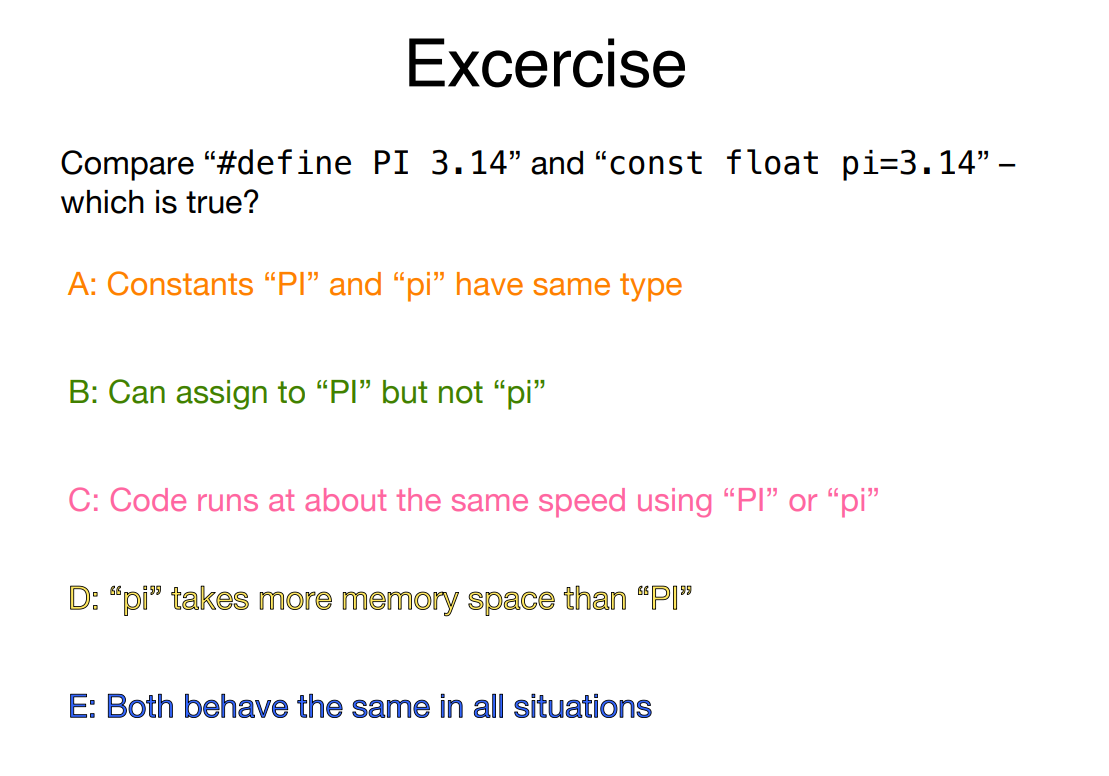
PI: no type.
2.2. Pointer
Just remember to initialize it, or it can point to “garbage”.
2.3. Array
The difference between char string1[] = "abc"
, char string2[3] = "abc"
and char *string3 = "abc"
.
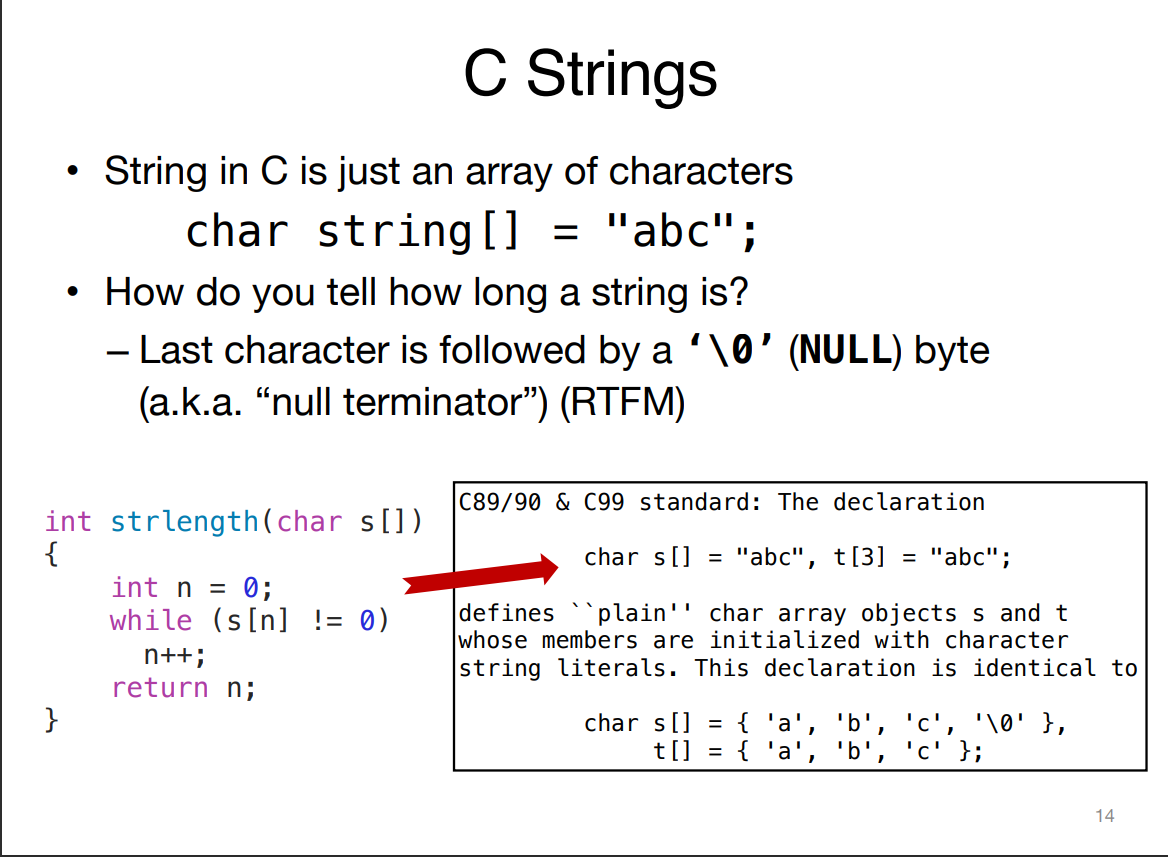
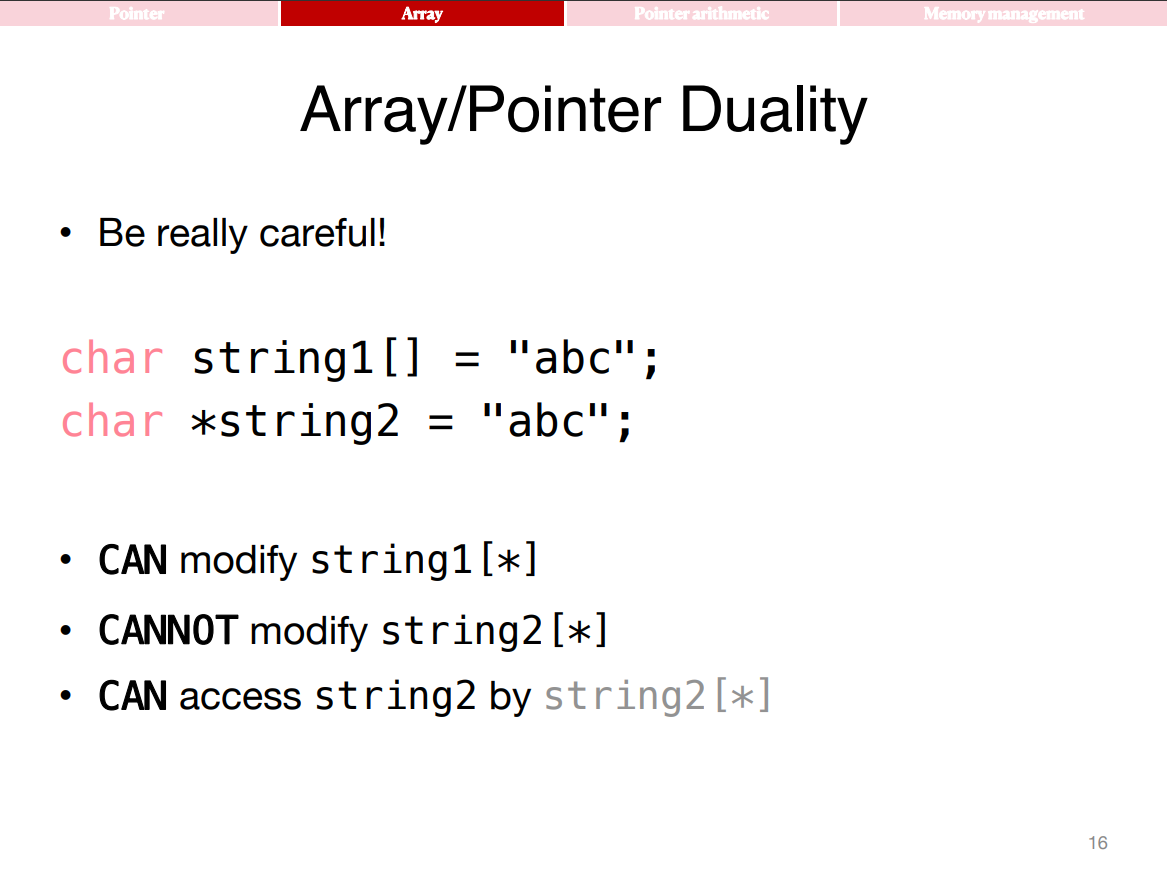
string1 ends with '\0', string3 is only readable
2.3.1. Further explanations:
-
What do these below mean? Be really careful!
1
2
3
4
5
6int arr[] = [ 3,5,6,7,9] ;
int *p = arr;
int (*p1)[5] = &arr; // p is a pointer, including 5 int elements, refering to arr.
int *p2[5]; // p2 is an array, including 5 int pointer.
int (*p)(void); // p is a pointer pointing to a function which accepts nothing.
int (*func arr[5])(float x); // func_arr is an array, including 5 function pointers, and they all accept float. -
Be carefull abt pointer in function
void inc_ptr(int *p) { p = p + 1; }
void inc_ptr(int **h){ *h = *h + 1; }
int A[3] = {50, 60, 70};
int A[3] = {50, 60, 70};
int *q = A;
int *q = A;
inc_ptr( q);
inc_ptr(&q);
printf( "q = %d\n",*q)
printf( "q = %d\n",*q)
- q = 50
- q = 60
2.3.2. Point past end of array
- Array size n; want to access from 0 to n-1, but test for exit by comparing to address one element past the last member of the array.
1 |
|
- C defines that one element past end of array must be a valid address, i.e., not causing an error
3. C Memory Managemen
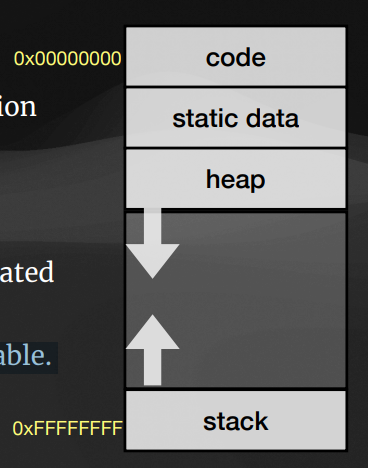
- Stack: automatically resize itself upon function invocations. (StackOverflow)
- Heap: this part is used for dynamic memory allocation. (malloc, free)
- Static Data: Global and static variables, allocated in compile time.
- Code: Contains code of the program, immutable. Also called text segment.
3.1. Where are Variables Allocated?
-
If declared outside a function, allocated in static storage
-
If declared inside function, allocated on the stack and freed when function returns
main()
is treated like a function
-
For the above two types, the memory management is automatic
- Don’t need to deallocating when no longer using them
- A variable does not exist anymore once a function ends!
4. RISC-V
Seek for more details: RISC-V Green_card
5. Combinatorial Logic
- Be careful:
$$
\begin{equation}
\begin{gathered}
A = A + A\overline{D}\\
\text{DeMorgan’s Law: } \overline{AB} = \overline{A} + \overline{B}, \overline{A+B} = \overline{A}\ \overline{B}
\end{gathered}
\end{equation}
$$
6. Sequence Logic
- Some terminology:
- Clk-to-q: (t_prop) is the time for a signal to propagate through a flip-flop.
- Propagation delay: (t_combinational) is the longest delay for any combinational logic (which by definition is surrounded by two flip-flop)
- Setup time: (t_setup) is the time before the rising clock edge that the input to a flip-flop must be valid.
- Hold time: The minimum time during which the input must be valid after the clock edge
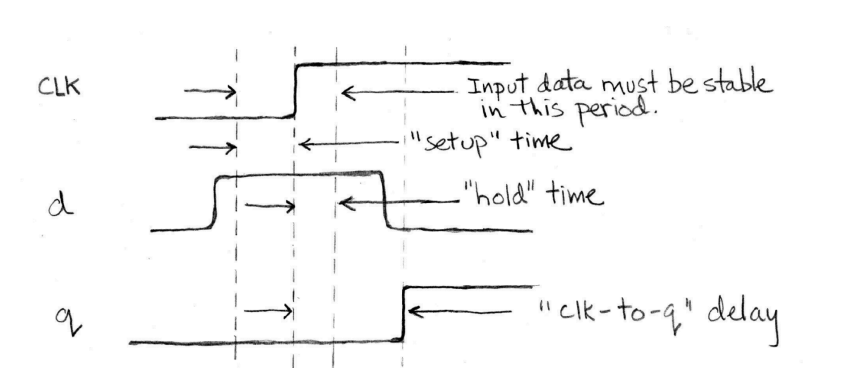
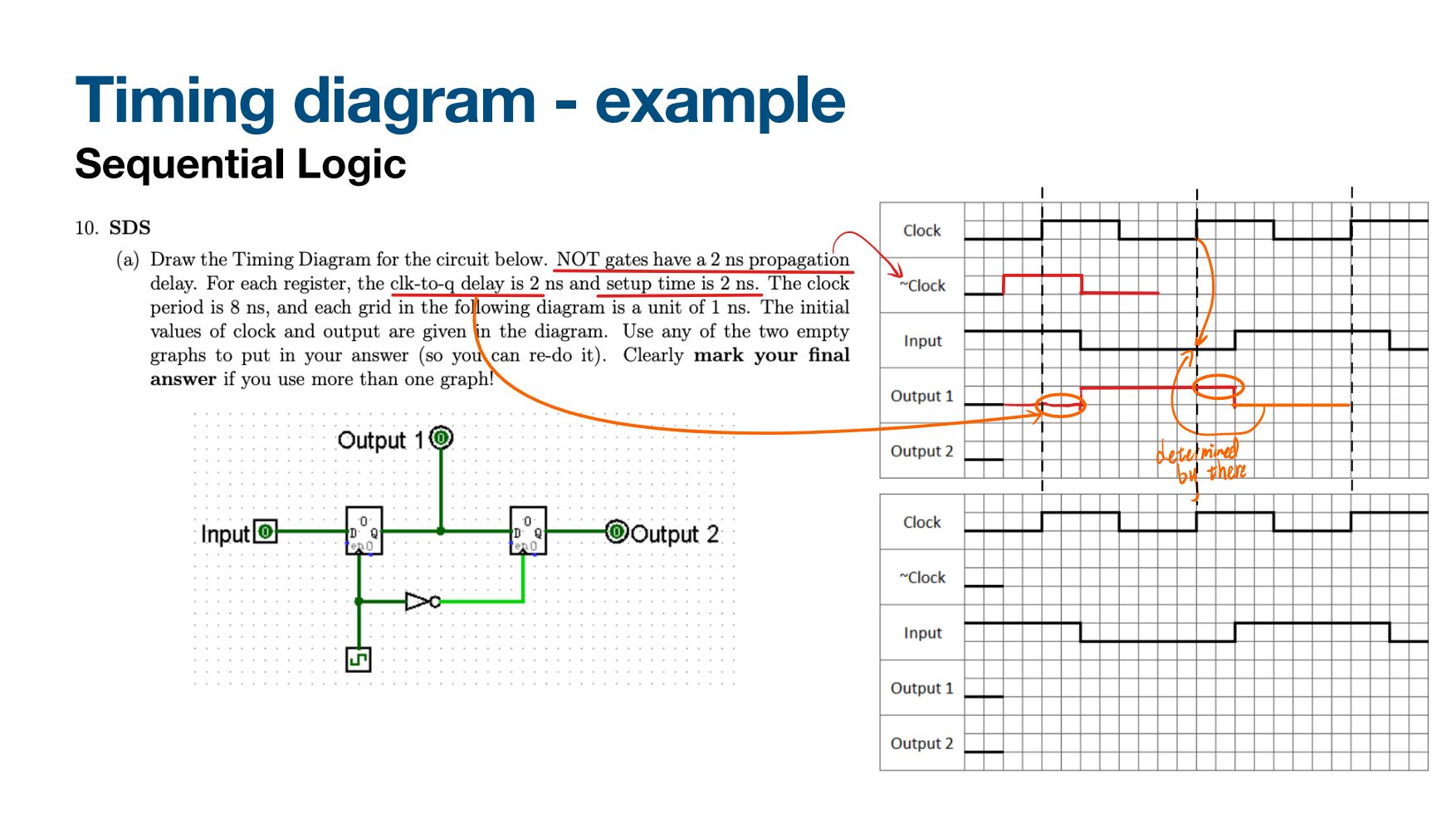
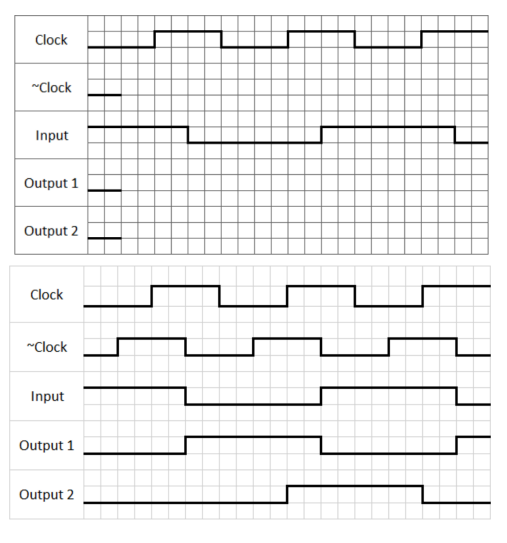
Therefore, to be more SPECIFIC:
Clk-to-q: when there is a rising edge, output propagate this time.
Setup time & Hold time, during which the input should be stable.
Also note that:
Combinatorial logic also has delays.
(Setup time violation) In a SDS, a system the clock period (or cycle time) must be at least as large as
$$
\begin{equation}
\begin{gathered}
t_{\text{prop}}(t_{\text{clk-to-q}})+t_{\text{combinational}}+t_{\text{setup}}\leq \text{min clock period}\\
\text{Max frequency = 1/min clock period}
\end{gathered}
\end{equation}
$$
(Hold time violation) In a feedback SDS(circuits contain register to register propagation), the hold time must not exceed:
$$
\text{hold time}\leq t_{\text{prop}}(t_{\text{clk-to-q}})+t_{\text{combinational}}
$$
E.G
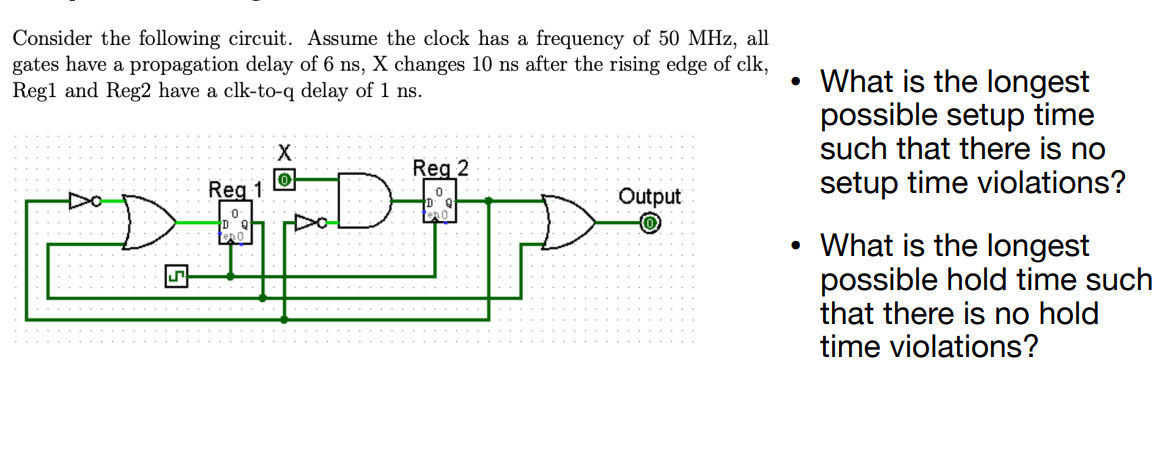
Solution:
Setup time: the critical path of “outer” combinational circuit.
Hold time: The shortest path including self register.
ATT: Evaluate each register separately
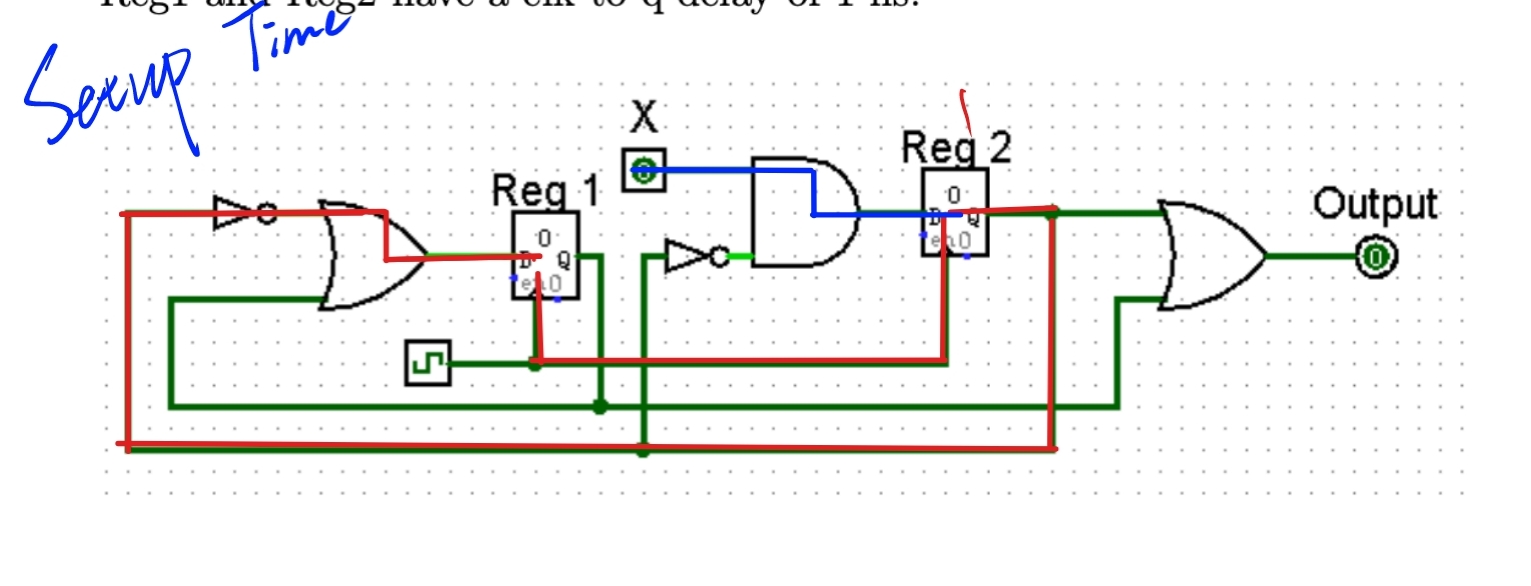
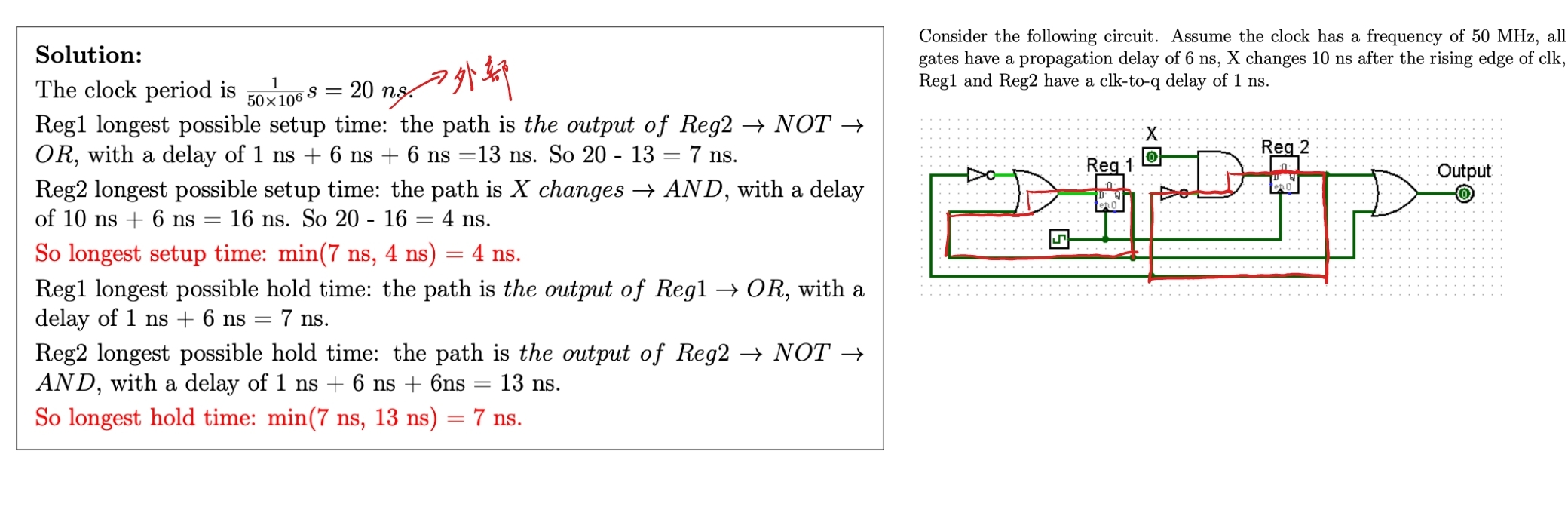